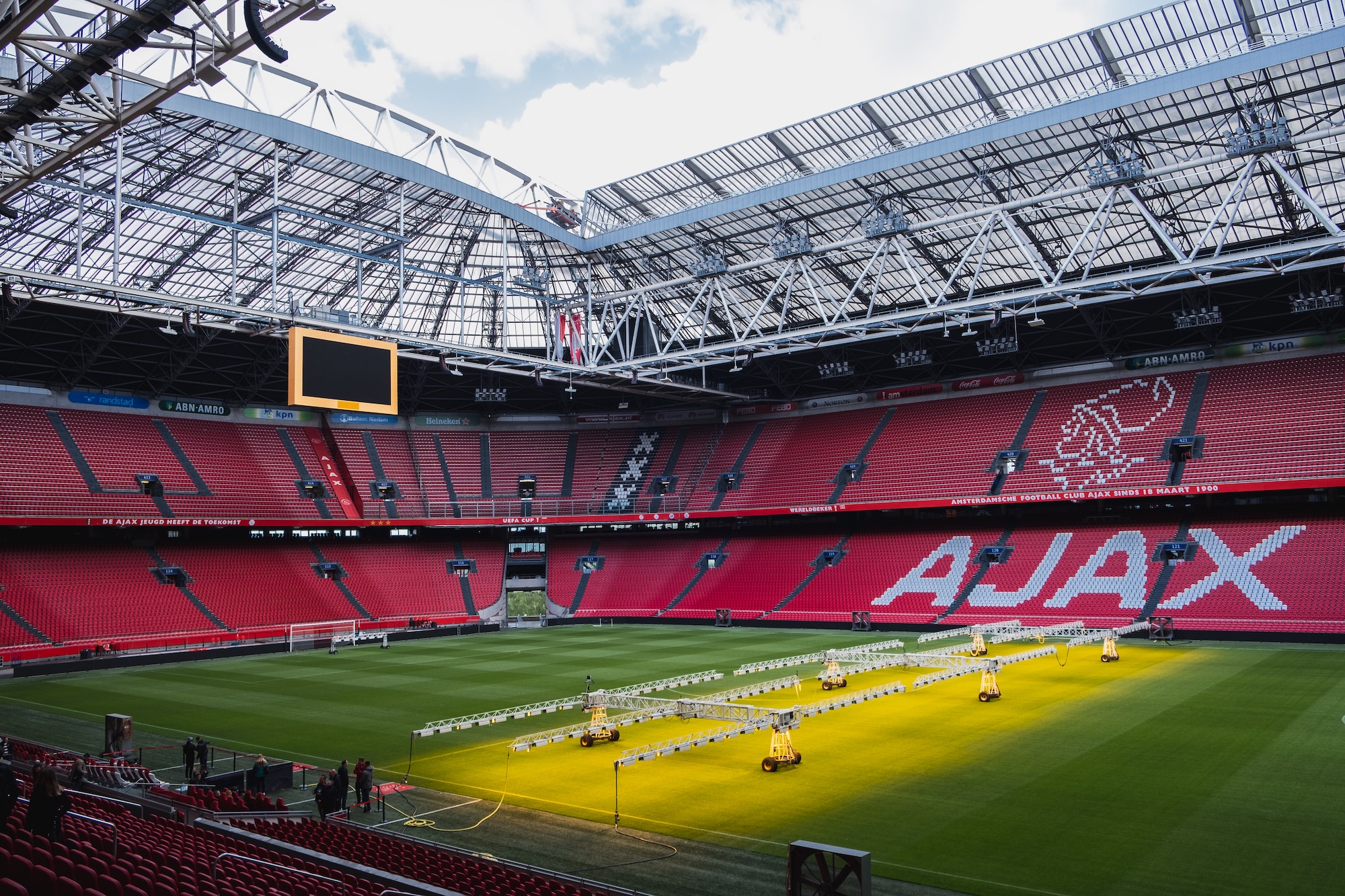
New Drupal contrib module: Browser Storage Commands
This week, I had a need for storing information in browser storage as a user goes through a series of modal forms. I couldn't use PrivateTempStore in this case as I didn't want to store the information in the database. I was also using Drupal modals with AJAX callbacks and realized that there aren't AJAX commands provided by core that will write to the browser storage. It's not a terribly complex problem, but was lacking an implementation in contrib space. So I went ahead and created a new contrib module that makes it simple to use AJAX commands to add or delete from local or session storage.
If you find yourself wanting to use browser storage, of course you could just use straight JavaScript and do localStorage.setItem(), etc to your heart's content. But when you're dealing with AJAX forms, it can be nice to be able to just add an AJAX command to the AjaxResponse and be done with it. If you're in a similar boat, try out my module!
If you are unfamiliar with how AJAX commands work, here is a brief overview (you could also just browse the code).
First, you need the PHP code that defines a command class that implements CommandInterface. The only requirement for this class is that it implements the render() function. Here is an example:
/**
* {@inheritdoc}
*/
public function render() {
return [
'command' => 'storageAdd',
'key' => $this->key,
'data' => $this->data,
'storage' => $this->storage,
];
}
It just needs to return an array. The 'command' element needs to be a unique command name for this specific command. It needs to match the function that we'll be adding in JavaScript below. The other array elements can be whatever you want that needs to be passed to the JavaScript function.
Next, create the JavaScript file. For every command, we need to add a function at: Drupal.AjaxCommands.prototype.<mycommand>. The function has three parameters: ajax, response, and status. The response parameter is the one that will contain the array values that the above render() function returned. The JavaScript will look like this:
Drupal.AjaxCommands.prototype.storageAdd = function (ajax, response, status) {
// Get the correct browser storage.
let storage = localStorage;
if (response.storage === 'session') {
storage = sessionStorage;
}
// Truncated...
// Save the new object.
storage.setItem(response.key, JSON.stringify(data));
}
That's basically it! This was just a brief overview, but if you want to go more in-depth, I'd recommend checking out this video:
- Log in to post comments